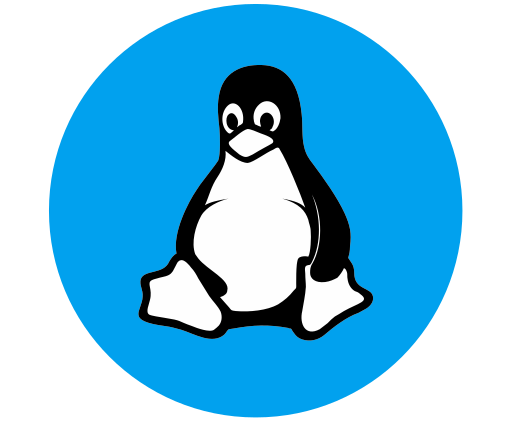
Linux Tutorials!
Why do I need this script?
In today’s IT environments, maintaining optimal disk usage is crucial to ensure system performance and prevent downtime. High disk usage can lead to a multitude of problems, including slow performance, system crashes, and data loss. To mitigate these risks, it’s essential to monitor disk usage regularly and take proactive measures.
Key Components of the Script
- Defining Email Addresses: At the beginning of the script, we define the email addresses for sending alerts. This includes the sender’s email and the recipient’s email.
FROM_EMAIL="email1@domain.com"
TO_EMAIL="email2@domain.com"
- Setting the Threshold: We set the threshold for disk usage. In this example, the threshold is set to 85%, but you can adjust it according to your requirements.
THRESHOLD=85
- Fetching Disk Usage Information: The script fetches the current disk usage percentage, disk name, hostname, IP address, and available disk memory.
DISK_USAGE=$(df -h / | awk 'NR==2 {print $5}' | sed 's/%//')
DISK_NAME=$(df -h / | awk 'NR==2 {print $1}' | sed 's/%//')
HOSTNAME=$(hostname)
IP=$(hostname -I | awk '{print $1}')
- Logging and Alerting: If the disk usage exceeds the threshold, the script logs the information and sends an alert email to the specified recipient.
if [ "$DISK_USAGE" -gt "$THRESHOLD" ]; then
LOG_FILE="/opt/scripts/log/disk_usage.log"
TIMESTAMP=$(date "+%Y-%m-%d %H:%M:%S")
MESSAGE="Disk usage on server ${HOSTNAME} (IP:${IP}), disk name ${DISK_NAME} (capacity: ${DISK_MEMORY_CAPACITY}, free space: ${DISK_MEMORY_FREE}, used: ${DISK_MEMORY_USED}) is above ${THRESHOLD}%! Current usage is ${DISK_USAGE}%. Script executed at ${TIMESTAMP}."
echo "$MESSAGE" >> "$LOG_FILE"
subject="ALERT! Service: check_disk - $(date +%Y-%m-%d)"
echo "$MESSAGE" | mail -a "From: ${FROM_EMAIL}" -s "$subject" ${TO_EMAIL}
fi
My Script for Monitoring Disk Usage with Email Alert
- Create folders for the script and logging
mkdir -p /opt/scripts/log/
- Copy the content of the script in the file:
nano alert_mail_check_disk.sh
Content of the file:
#!/bin/bash
# Define email addresses
FROM_EMAIL="email1@domain.com"
TO_EMAIL="email2@domain.com"
# Set the threshold for disk usage (85% in this case)
THRESHOLD=85
# Get the current disk usage percentage for the root filesystem
DISK_USAGE=$(df -h / | awk 'NR==2 {print $5}' | sed 's/%//')
DISK_NAME=$(df -h / | awk 'NR==2 {print $1}' | sed 's/%//')
HOSTNAME=$(hostname)
IP=$(hostname -I | awk '{print $1}')
# Get the available disk memory
DISK_MEMORY_FREE=$(df -h | awk '$NF == "/" {print $4}')
DISK_MEMORY_USED=$(df -h | awk '$NF == "/" {print $3}')
DISK_MEMORY_CAPACITY=$(df -h | awk '$NF == "/" {print $2}')
DISK_MEMORY_USED_PROCENT=$(df -h | awk '$NF == "/" {print $5}')
# Check if disk usage exceeds the threshold
if [ "$DISK_USAGE" -gt "$THRESHOLD" ]; then
# Log the information
LOG_FILE="/opt/scripts/log/disk_usage.log"
TIMESTAMP=$(date "+%Y-%m-%d %H:%M:%S")
MESSAGE="Disk usage on server ${HOSTNAME} (IP:${IP}), disk name ${DISK_NAME} (capacity: ${DISK_MEMORY_CAPACITY}, free space: ${DISK_MEMORY_FREE}, used: ${DISK_MEMORY_USED}) is above ${THRESHOLD}%! Current usage is ${DISK_USAGE}%. Script executed at ${TIMESTAMP}."
echo "$MESSAGE" >> "$LOG_FILE"
subject="ALERT! Service: check_disk - $(date +%Y-%m-%d)"
echo "$MESSAGE" | mail -a "From: ${FROM_EMAIL}" -s "$subject" ${TO_EMAIL}
fi
- Make a file executable:
chmod +x alert_mail_check_disk.sh
Crontab Installation
- Edit the crontab to execute script automaticaly:
crontab -e
- Add the following line in the Crontab to check disk usage once per day at 22.00h
# Check disk usage every day at 22.00h
00 22 * * * bash /opt/scripts/alert_mail_check_disk.sh
If you want to do more checks during the day, you can do it by changing the parameters. Check the following link to create your own schedule.
Example to check disk usage every 15 Minutes:
# Check disk usage every 15 Minutes
*/15 * * * * bash /opt/scripts/alert_mail_check_disk.sh