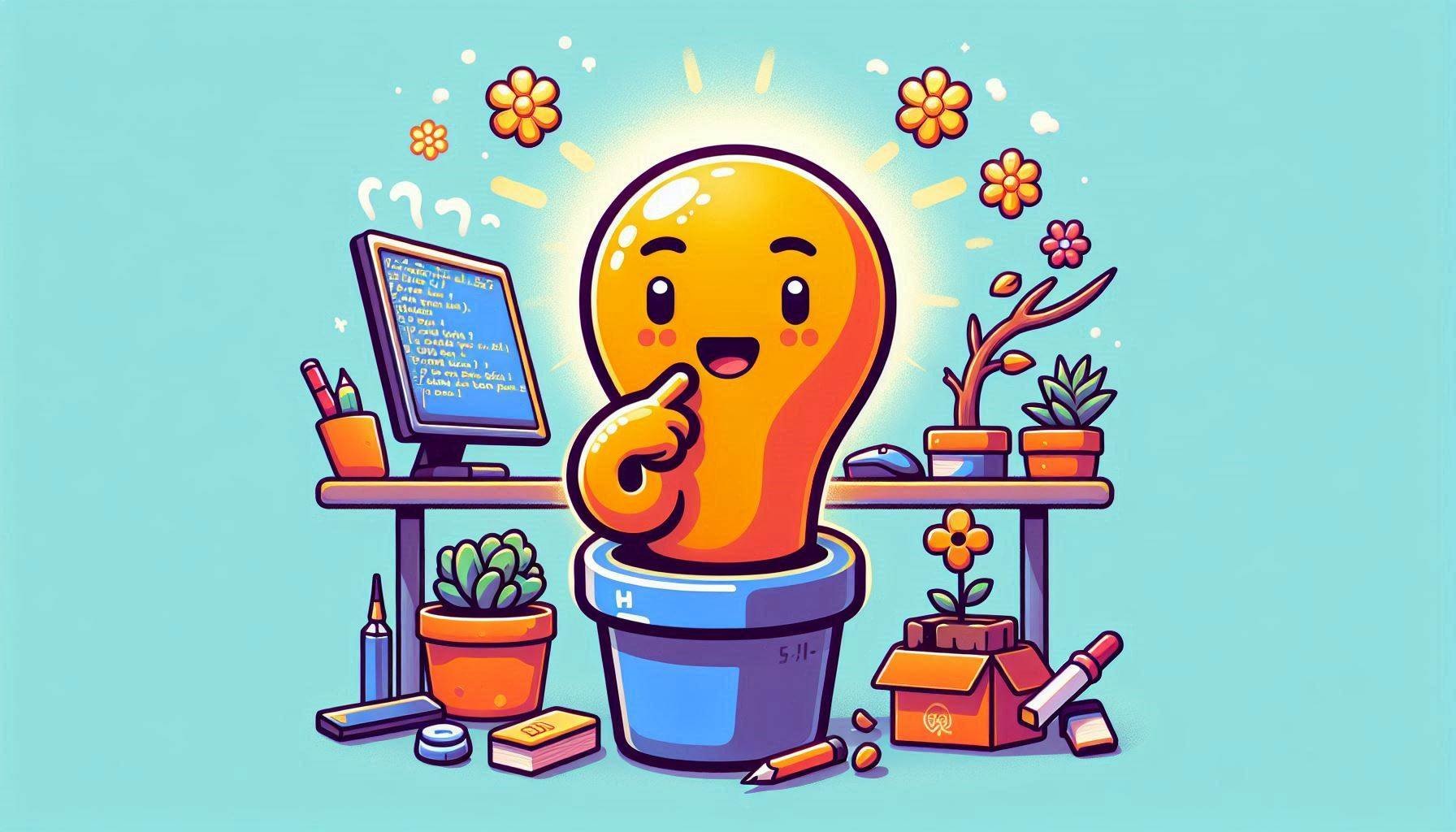
Learn Bash with Our Tutorials!
Introduction
Bash handles maths operations differently from many other programming languages.
In this article, you will see some common numerical operations.
More information about floating-point arithmetic you can find at the link.
Arithmetic Operations
num1=5
num2=3
sum=$((num1 + num2))
echo $sum # Output: 8
Increment and Decrement
num=5
((num++))
echo $num # Output: 6
((num--))
echo $num # Output: 5
Comparison
num1=5
num2=3
if [ $num1 -gt $num2 ]; then
echo "$num1 is greater than $num2"
else
echo "$num1 is not greater than $num2"
fi
Floating Point Arithmetic
Bash does not support floating-point arithmetic natively, but you can use external tools like bc
or awk
.
num1=5.5
num2=3.2
result=$(echo "$num1 + $num2" | bc)
echo $result # Output: 8.7
Practical Examples
Counting Words in a String
str="Hello World from Bash"
word_count=$(echo $str | wc -w)
echo "Word count: $word_count" # Output: Word count: 4
Calculating the Sum of Numbers in a File
sum=0
while read num; do
sum=$((sum + num))
done < numbers.txt
echo "Sum: $sum"