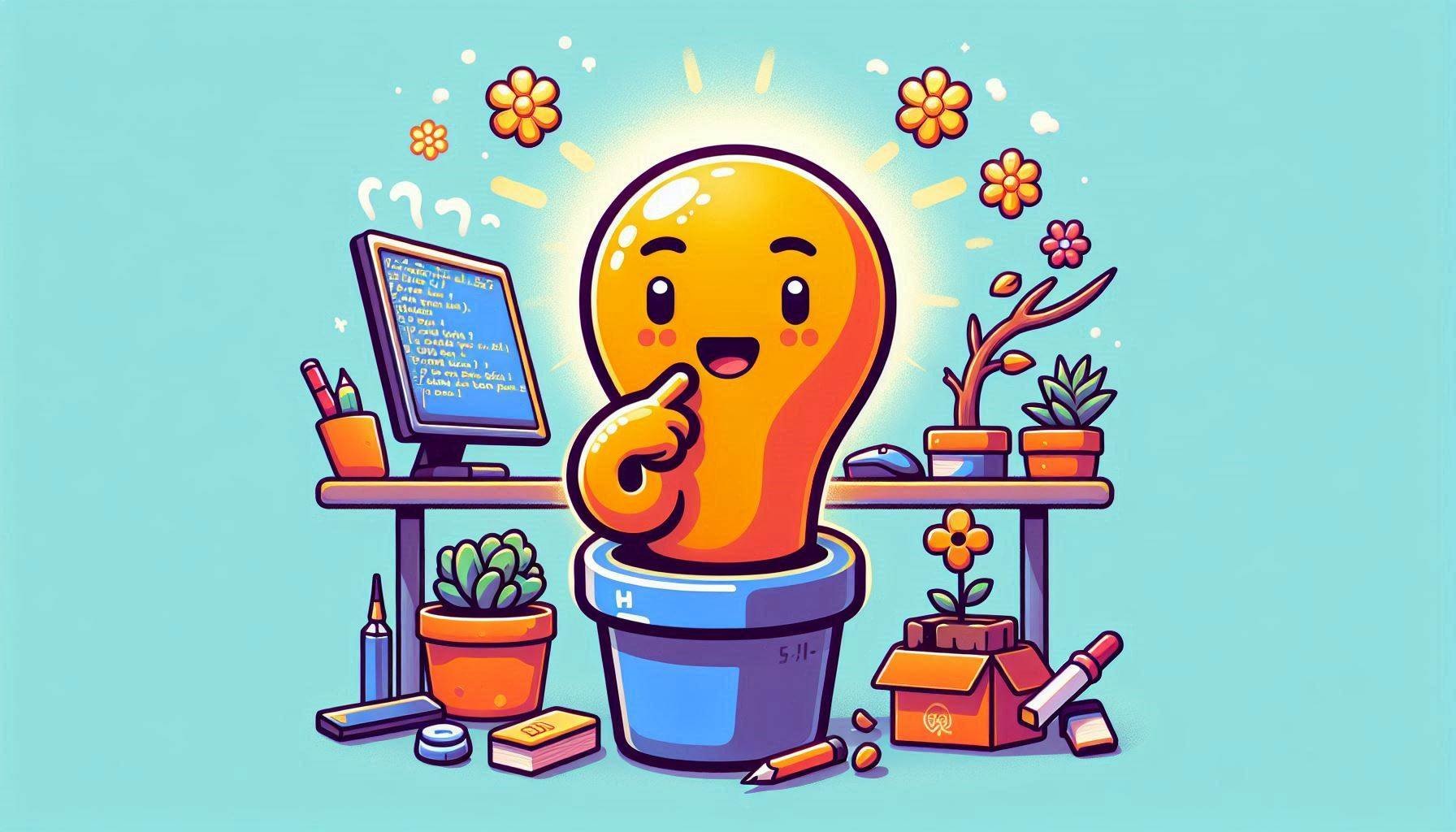
Learn Bash with Our Tutorials!
Bash functions are a powerful feature that allows you to create reusable blocks of code within your scripts. By using functions, you can make your scripts more modular, easier to read, and maintain. In this article, we’ll explore how to define and use Bash functions, along with some practical examples.
What are Bash Functions?
A Bash function is a block of code that you can define once and call multiple times within your script. Functions help you avoid code duplication and make your scripts more organized.
Defining a Function
To define a function in Bash, you use the following syntax:
function_name() {
commands
}
Alternatively, you can use the function
keyword:
function function_name {
commands
}
Calling a Function
Once you’ve defined a function, you can call it by simply using its name:
function_name
Function Parameters
You can pass parameters to a function just like you would with a script. Inside the function, you can access these parameters using $1
, $2
, etc.
greet() {
echo "Hello, $1!"
}
greet "World"
Returning Values
Functions can return values using the return
statement, which returns an exit status (0-255). For more complex return values, you can use echo or global variables.
add() {
local sum=$(( $1 + $2 ))
echo $sum
}
result=$(add 5 3)
echo "The sum is: $result"
Practical Examples
- A function to check if a file exists:
file_exists() {
if [ -f "$1" ]; then
echo "File exists."
else
echo "File does not exist."
fi
}
file_exists "/path/to/file"
- A function to calculate factorial:
factorial() {
if [ $1 -le 1 ]; then
echo 1
else
local temp=$(( $1 - 1 ))
local result=$(factorial $temp)
echo $(( $1 * result ))
fi
}
echo "Factorial of 5 is: $(factorial 5)"