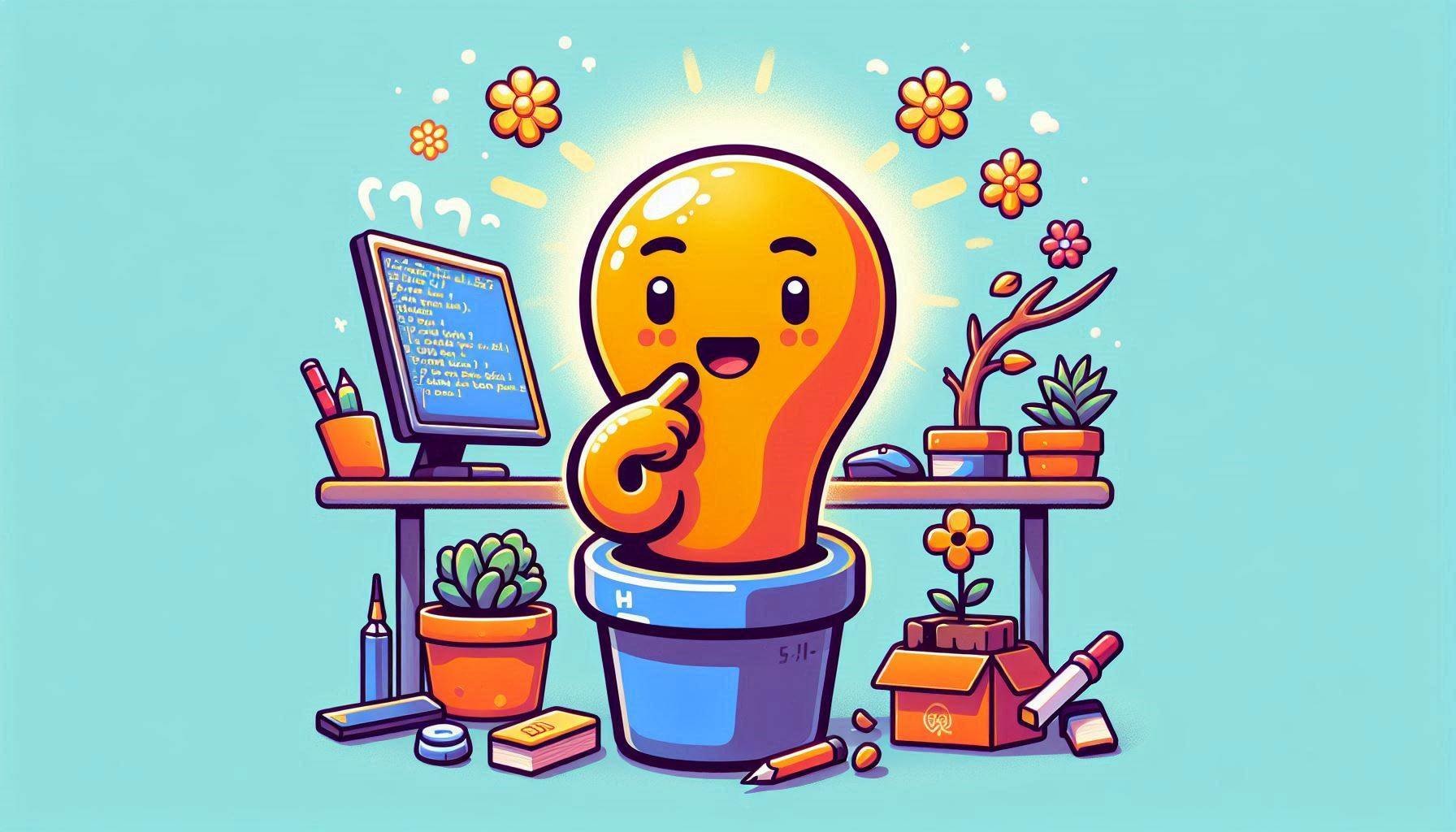
Learn Bash with Our Tutorials!
Bash command substitution is a feature that allows you to execute a command and replace it with its output. This can be incredibly useful for scripting and automating tasks in a Unix-like environment. In this article, we’ll explore how to use command substitution, its syntax, and some practical examples to help you get started.
What is Command Substitution?
Command substitution enables you to capture the output of a command and use it as an argument in another command.
This can be done using two different syntaxes:
- Backticks (
result=`command`
- Dollar sign and parentheses ($()):
result=$(command)
The second syntax is preferred because it is more readable and can be nested.
Basic Examples
Using date
command:
current_date=$(date)
echo "Today's date is: $current_date"
Using ls
command:
files=$(ls)
echo "Files in the current directory: $files"
Nested Command Substitution
One of the advantages of using the $()
syntax is that it allows for nesting. Here’s an example:
nested_result=$(echo $(date))
echo "Nested command substitution result: $nested_result"
Output:
Nested command substitution result: Thu Jul 25 09:14:58 AM UTC 2024
Practical Applications
Command substitution can be used in various scenarios to make your scripts more efficient:
- Capturing command output for conditional statements:
if [ $(whoami) = 'root' ]; then
echo "You are the root user."
else
echo "You are not the root user."
fi
- Using command output in loops:
for file in $(ls); do
echo "Processing file: $file"
done