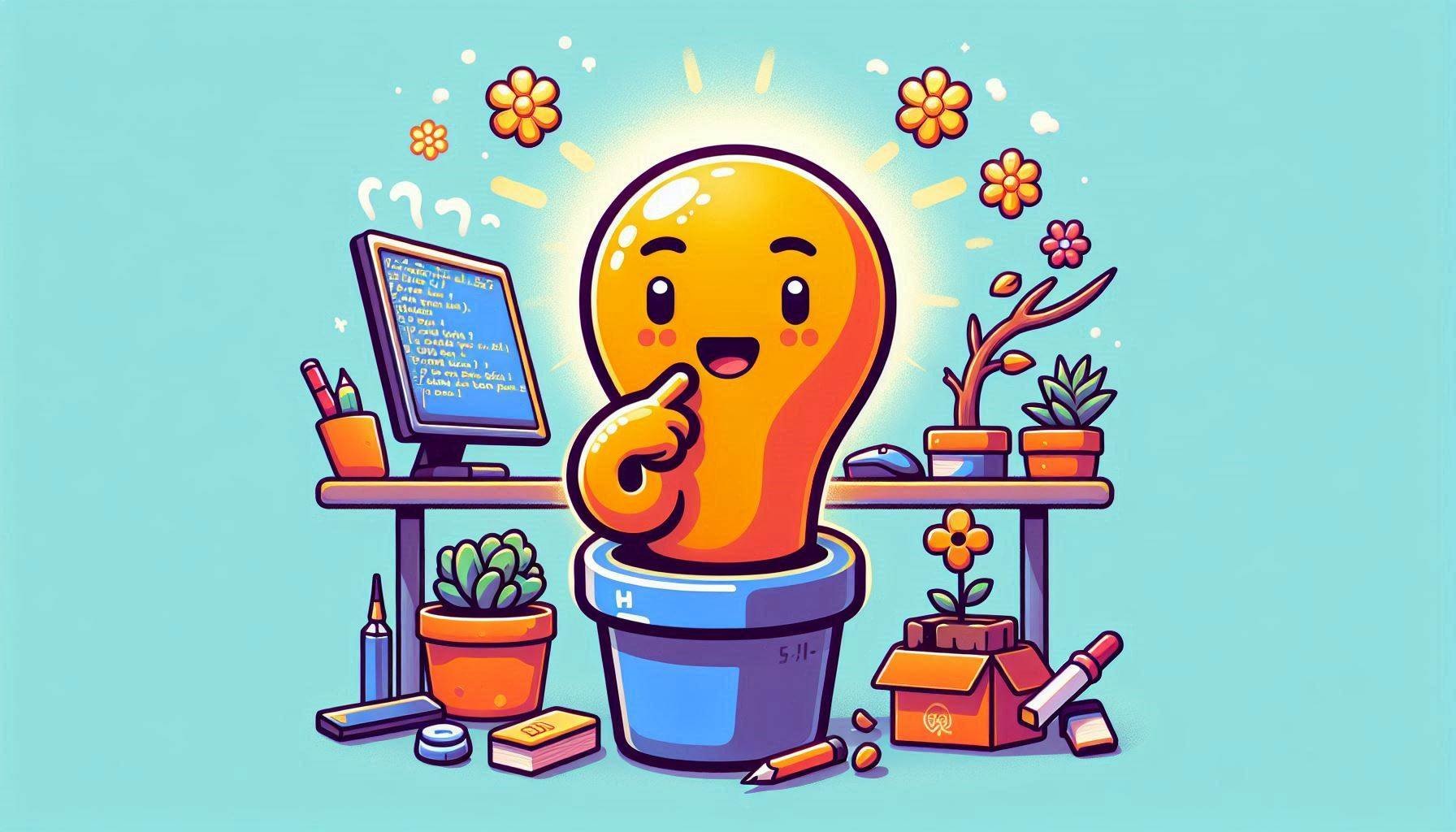
Learn Bash with Our Tutorials!
One essential aspect of scripting is the ability to interact with users by reading user input. This article will guide you through the basics of reading user input in Bash, including using the read
 command and handling input effectively.
Using the read
Command
The read
command is the primary method for capturing user input in Bash. It reads a line of text from standard input and assigns it to a variable.
Basic syntax
read variable_name
Example
echo "Enter your name:"
read name
echo "Hello, $name!"
In this example, the script prompts the user to enter their name and then greets them.
Reading Multiple Inputs
You can also read multiple inputs in a single line by specifying multiple variable names:
echo "Enter your first and last name:"
read first_name last_name
echo "Hello, $first_name $last_name!"
Using Flags with read
The read
command comes with several useful flags:
-p
allows you to specify a prompt.-s
hides the input (useful for passwords).-t
sets a timeout for input.
Example
read -p "Enter your username: " username
read -s -p "Enter your password: " password
echo
echo "Username: $username"
echo "Password: $password"
In this example, the script prompts the user for their username and password, hiding the password input for security.
Handling Input with Default Values
You can provide default values for user input by using parameter expansion:
read -p "Enter your favorite color [blue]: " color
color=${color:-blue}
echo "Your favorite color is $color."